平常在微博也就看看热搜,别的都用不到,搞了个这个服务卡片,自己用着舒服(手动狗头)
HarmonyOS - 开发一个微博热搜服务卡片
效果图
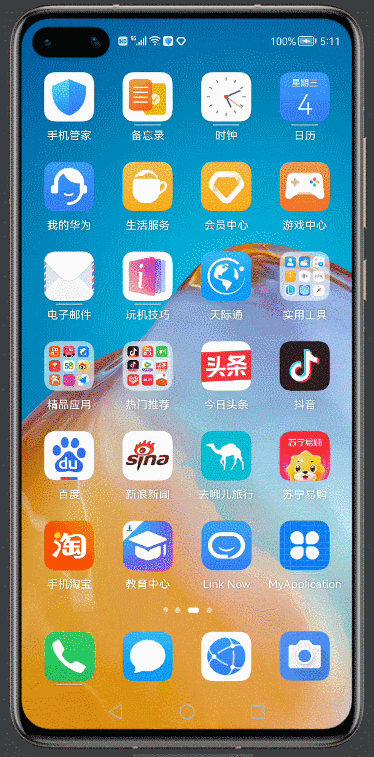
关键原理
使用JS的服务卡片,相对于Java的服务卡片,JS的服务卡片的支持更加齐全,效果更佳
使用了JS的UI中的List组件显示微博的热搜列表,每个子项包括序号、标题、热度和类型
通过在Ability的onCreateForm(Intent intent)函数中进行热搜的数据分析返回
使用Java的Jsoup包进行网页的html数据获取,再使用其分析出微博的热搜信息
服务卡片的页面和样式
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <list class="list"> <list-item class="list-item"> <div class="div"> <text class="item_number" style="color: #999;" >序号</text> <text class="item_title" style="color: #999;">关键词</text> </div> </list-item> <list-item for="{{cards}}" class="list-item"> <div class="div"> <text class="item_number">{{ $item.number }}</text> <text class="item_title">{{ $item.title }}</text> <text class="item_count">{{ $item.count }}</text> <text class="item_type" style="background-color: {{$item.color}};" >{{ $item.type }}</text> </div> </list-item> </list>
|
然后是样式文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
| .list{ align-items:center; }
.list-item{ border-radius: 10px; background-color: #f2f2f2; margin-bottom: 3px; }
.div{ margin:6px; }
.item_number { font-size: 12px; color: #f26d5f; width: 30px; text-align: left; }
.item_title{ font-size: 12px; color: #0078b6; width: 220px; text-align: left; }
.item_count{ font-size: 12px; text-align: right; width: 60px; color: #808080; }
.item_type{ margin-left: 5px; font-size: 12px; text-align: right; width: 15px; color: #fff; border-radius: 2px; }
|
接着是js文件
1 2 3 4 5 6 7 8 9 10 11 12 13
| export default { data: { cards: [ { "number": 1, "title": "义乌1秒发6只冠军同款 ", "count": 4591790, "type": "新", "color": "#f2f2f2" } ] } }
|
Java端进行网页请求和分析出微博热搜信息
首先需要引入一个包Jsoup,含有网页请求和网页分析的工具
1
| compile 'org.jsoup:jsoup:1.12.1'
|
然后写一个获取微博热搜的工具类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| import org.jsoup.Jsoup; import org.jsoup.internal.StringUtil; import org.jsoup.nodes.Document; import org.jsoup.nodes.Element; import org.jsoup.select.Elements;
import java.io.IOException; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map;
public class GetWeiboListUtil {
private static final String URL = "https://s.weibo.com/top/summary?cate=realtimehot";
public static List<Map<String, String>> getWeiboList() { List<Map<String, String>> weiboList = new ArrayList<>(); try { Document document = Jsoup.connect(URL).get(); Element plTopRealTimeHot = document.getElementById("pl_top_realtimehot"); Elements tBody = plTopRealTimeHot.children().get(0).children().get(1).children(); for (Element element : tBody) { String number = element.children().get(0).text(); if (StringUtil.isBlank(number)) { number = "↑"; } String title = element.children().get(1).children().get(0).text(); String count = ""; if (element.children().get(1).children().size() > 1) { count = element.children().get(1).children().get(1).text(); } String type = element.children().get(2).text(); String color = ""; if (!StringUtil.isBlank(type)) { switch (type) { case "热": color = "#ff9406";break; case "新": color = "#ff3852";break; case "商": color = "#00b7ee";break; } } Map<String, String> weiboItem = new HashMap<>(); weiboItem.put("number", number); weiboItem.put("title", title); weiboItem.put("count", count); weiboItem.put("type", type); weiboItem.put("color", color); weiboList.add(weiboItem); } } catch (IOException e) { e.printStackTrace(); } return weiboList; } }
|
然后是实现Ability的创建事件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55
| import ***.GetWeiboListUtil; import ohos.aafwk.ability.AbilitySlice; import ohos.aafwk.ability.FormBindingData; import ohos.aafwk.ability.ProviderFormInfo; import ohos.ace.ability.AceAbility; import ohos.aafwk.content.Intent; import ohos.utils.zson.ZSONArray; import ohos.utils.zson.ZSONObject;
import java.util.ArrayList; import java.util.List;
public class MainAbility extends AceAbility {
private static final String LIST_NAME = "cards"; private static final int DEFAULT_DIMENSION_4X4 = 4;
@Override public void onStart(Intent intent) { super.onStart(intent); }
@Override public void onStop() { super.onStop(); }
@Override protected ProviderFormInfo onCreateForm(Intent intent) { String formName = intent.getStringParam(AbilitySlice.PARAM_FORM_NAME_KEY); int dimension = intent.getIntParam(AbilitySlice.PARAM_FORM_DIMENSION_KEY, DEFAULT_DIMENSION_4X4); if (formName.equals("widget") && dimension == DEFAULT_DIMENSION_4X4) { ProviderFormInfo providerFormInfo = new ProviderFormInfo(); ZSONObject zsonObject = new ZSONObject(); List<Object> weiboList = new ArrayList<>(GetWeiboListUtil.getWeiboList()); ZSONArray zsonArray = new ZSONArray(weiboList); zsonObject.put(LIST_NAME, zsonArray); providerFormInfo.setJsBindingData(new FormBindingData(zsonObject)); return providerFormInfo; } return null; }
@Override protected void onUpdateForm(long formId) { super.onUpdateForm(formId); }
@Override protected void onDeleteForm(long formId) { super.onDeleteForm(formId); } }
|
此为博主副博客,留言请去主博客,转载请注明出处:https://www.baby7blog.com/myBlog/106.html